The following Apex code demonstrates how to generate XML containing the Salesforce account information along with its related Contacts. The code first adds the Account details within the root tag of the XML, followed by a list of Contacts inside a <Contacts>
tag.
Account acc = [SELECT Id, Name, Type, (SELECT FirstName, MiddleName, LastName, Email, Phone, BirthDate, MailingStreet, MailingCity, MailingState, MailingPostalCode, MailingCountry FROM Contacts) FROM Account limit 1];
// Create the root XML document
DOM.Document doc = new DOM.Document();
// Create root element
DOM.XmlNode root = doc.createRootElement('AccountInfo', null, null);
root.addChildElement('Name', null, null).addTextNode(acc.Name);
string account_type = acc.Type == NULL ? '' : acc.Type;
root.addChildElement('Type', null, null).addTextNode(account_type);
DOM.XmlNode ContactsNode = root.addChildElement('Contacts', null, null);
// Add Contacts
for (Contact cont: acc.Contacts) {
String birthdate = '';
if(cont.BirthDate != NULL){
DateTime dt = DateTime.newInstance(cont.BirthDate.year(), cont.BirthDate.Month(), cont.BirthDate.Day());
birthdate = dt.format('YYYY-MM-dd');
}
String contact_id = cont.Id;
String contact_firstname = cont.FirstName != NULL ? cont.FirstName : '';
String contact_middlename = cont.MiddleName != NULL ? cont.MiddleName : '';
String contact_lastname = cont.LastName;
String contact_email = cont.Email != NULL ? cont.Email : '';
DOM.XmlNode contactNode = ContactsNode.addChildElement('Contact', null, null);
contactNode.setAttribute('xmlns:xsi', 'http://www.w3.org/2001/XMLSchema-instance');
// Customer details
contactNode.setAttribute('Type', 'applicant');
contactNode.addChildElement('CustomerID', null, null).addTextNode(contact_id);
DOM.XmlNode nameNode = contactNode.addChildElement('Name', null, null);
nameNode.addChildElement('FirstName', null, null).addTextNode(contact_firstName);
nameNode.addChildElement('MiddleName', null, null).addTextNode(contact_middleName);
nameNode.addChildElement('LastName', null, null).addTextNode(contact_lastname);
contactNode.addChildElement('Email', null, null).addTextNode(contact_email);
String StreetAddress = cont.MailingStreet == NULL ? '' : cont.MailingStreet;
String city = cont.MailingCity == NULL ? '' : cont.MailingCity;
String state = cont.MailingState == NULL ? '' : cont.MailingState;
String postalcode = cont.MailingPostalCode == NULL ? '' : cont.MailingPostalCode;
String country = cont.MailingCountry == NULL ? '' : cont.MailingCountry;
DOM.XmlNode addressNode = contactNode.addChildElement('Address', null, null);
addressNode.setAttribute('Type', 'current');
addressNode.addChildElement('StreetAddress', null, null).addTextNode(StreetAddress);
addressNode.addChildElement('City', null, null).addTextNode(city);
addressNode.addChildElement('State', null, null).addTextNode(state);
addressNode.addChildElement('PostalCode', null, null).addTextNode(PostalCode);
addressNode.addChildElement('Country', null, null).addTextNode(Country);
}
System.debug(doc.toXmlString());
The XML tree is generated using the above code and a sample account record.
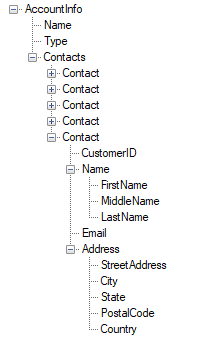
Then the following apex code showcases how to parse the generated XML to extract the Account and Contacts data.
Dom.Document docParser = new Dom.Document();
docParser.load(XMLString);
// Get the root element
Dom.XMLNode rootParser = docParser.getRootElement();
// Find the 'Response' node
for (Dom.XMLNode rootElement : rootParser.getChildElements()) {
system.debug(rootElement.getName()+':'+rootElement.getText());
if (rootElement.getName() == 'Contacts') {
Dom.XMLNode contactsNode = rootElement;
System.debug('contactsNode Name: ' + contactsNode.getName());
//loop through contact elements
for (Dom.XMLNode contNode : contactsNode.getChildElements()) {
system.debug(contNode.getName()+':'+contNode.getText());
if(contNode.getName() == 'Contact'){
for (Dom.XMLNode cont : contNode.getChildElements()) {
system.debug(cont.getName()+':'+cont.getText());
}
}
}
}
}