When attempting to display the HTML returned by an API in a Lightning Web Component (LWC), Salesforce security restrictions present some challenges. Specifically:
- LWC does not allow the use of the
srcdoc
attribute on<iframe>
elements due to security concerns. - Injecting full HTML content into a
<div>
usinginnerHTML
does not render properly when the HTML includes top-level tags like<html>
,<head>
, or<body>
. These tags are ignored by the browser in this context.
To work around these limitations, a Visualforce (VF) page is used to fetch the HTML from the external API. This VF page is then embedded within a parent Visualforce page via an <iframe>
. The outer VF page can then be added to the Lightning record page.
This approach ensures that when the page loads, the HTML is retrieved directly from the API and rendered correctly in the browser, without being affected by LWC’s content security restrictions.
STEP 1: Create an apex class to retrieve the HTML from the API endpoint so that it can be displayed on the VF page.
public class RetrieveHtmlFromApiController {
public String HtmlContent { get; set; }
public void loadContent() {
HtmlContent = geHtmlContent();
}
public static String geHtmlContent() {
try{
String Endpoint = 'https://example.com';
Http http = new Http();
HttpRequest request = new HttpRequest();
request.setEndpoint(Endpoint);
request.setMethod('GET');
HttpResponse response = http.send(request);
return response.getBody();
}catch(Exception ex){
return '<p style="color:red;">Error Fetching Map: '+ex.getMessage()+'</p>';
}
}
}
Make sure that the API endpoint URL is added to Remote Site Settings. For better security and manageability, it is recommended to use Named Credentials instead.
Step2: Create a Visualforce page to render the HTML returned from the above apex class.
Visualfroce Page Name: GetHtmlContent
<apex:page controller="RetrieveHtmlFromApiController" contentType="text/html" showHeader="false" sidebar="false" cache="false" action="{!loadContent}">
<apex:outputText value="{!HtmlContent}" escape="false" />
</apex:page>
Step 3: Create a main Visualforce page that embeds the Visualforce page from Step 2 using an <iframe>
.
This main page should be used when adding the component to Lightning record pages, Home pages, Screen Flows, and other Lightning contexts.
Visualforce Page Name: DisplayApiHtmlContent
<apex:page showHeader="false" sidebar="false" cache="false" contentType="text/html"
standardStylesheets="false" applyHtmlTag="false" applyBodyTag="false" docType="html-5.0">
<apex:slds />
<div class="slds-scope slds-p-around_medium">
<apex:form id="mapForm">
<apex:outputPanel id="mapBlock" layout="block">
<iframe
src="/apex/GetHtmlContent"
width="100%"
height="100%"
style="border: none;"
sandbox="allow-scripts allow-same-origin">
</iframe>
</apex:outputPanel>
</apex:form>
</div>
</apex:page>
Here is the outcome of the VF page.
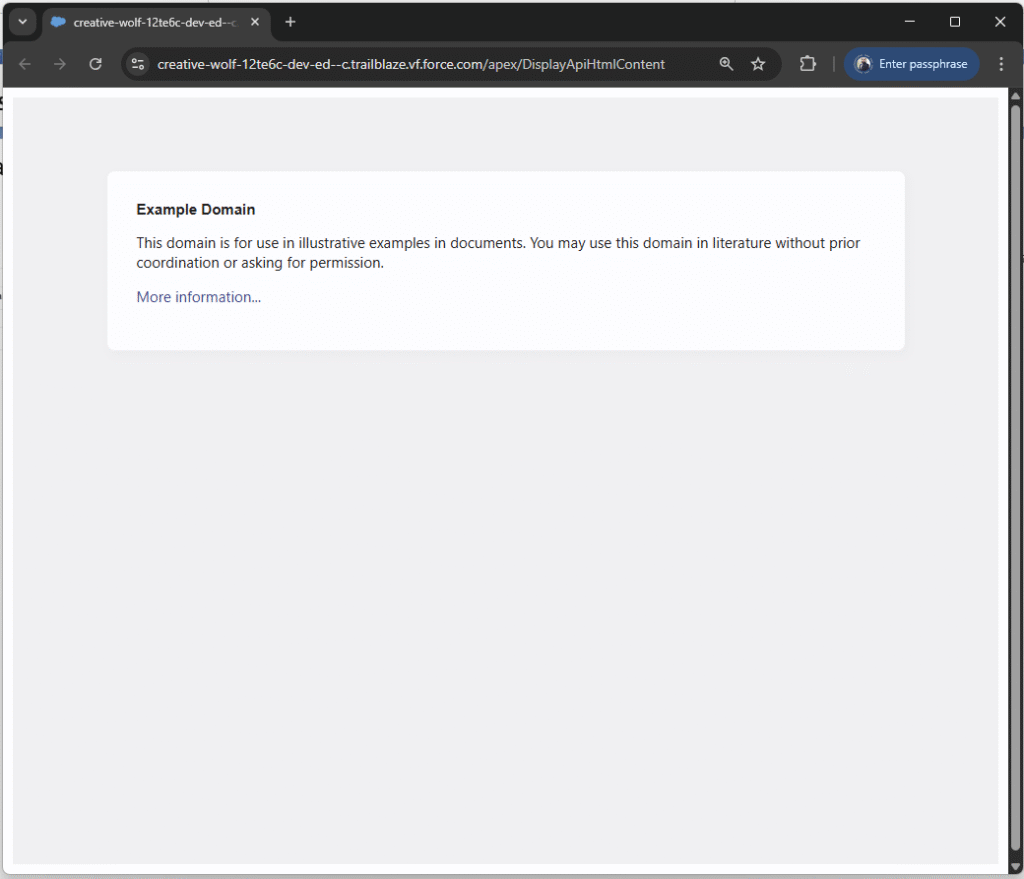