DESCRIPTION
The following apex invocable class/method takes the following inputs
- Stripe Customer Id
- Amount
- description
Then it posts a request to the stripe /v1/charges
endpoint. The charge object is used to charge a credit card or other payment source.
Get Stripe API Key
Users with Administrator permissions can access a Stripe account’s API keys by navigating to the Developers section of the Stripe dashboard and clicking on API Keys.
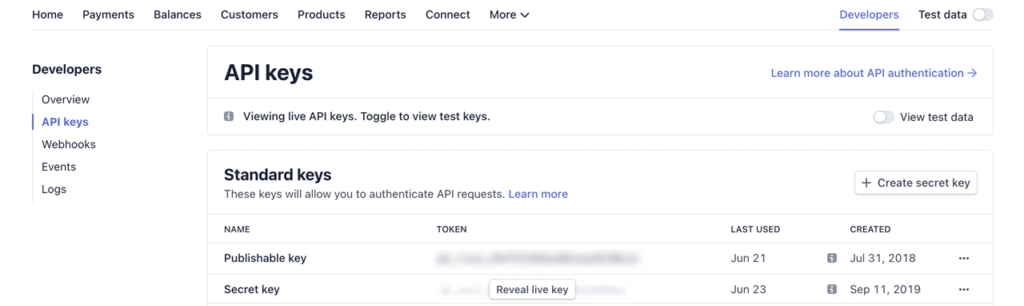
global class StripeChargeAPI{
private static final String EndPointURL = 'https://api.stripe.com/v1/charges';
@InvocableMethod(label='Create Stripe Charge' description='This method takes input from the flow and make a charge record on Stripe.')
public static void StriptCharge(list<CreateChargeRequest> Requests){
//Store the API key in the custom setting and then use custom setting here, so that you don't have to update the code, if the api key is expired.
String APIKey = 'sk_live_XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX';
for(CreateChargeRequest request :Requests){
CreateCharge(APIKey, Request.CustomerId, Request.Amount, Request.description, Request.statement_descriptor, Request.RecordId);
}
}
@future(callout=true)
public static void CreateCharge(String APIKey, String CustomerId, decimal Amount, String description, String statement_descriptor, String RecordId){
Amount = Math.round(Amount * 100);
HttpRequest http = new HttpRequest();
http.setEndpoint(EndPointURL);
http.setMethod('POST');
Blob headerValue = Blob.valueOf(APIKey + ':');
String authorizationHeader = 'BASIC ' +
EncodingUtil.base64Encode(headerValue);
http.setHeader('Authorization', authorizationHeader);
http.setBody('amount='+amount + '¤cy=USD&description='+EncodingUtil.urlEncode(description,'UTF-8')+'&statement_descriptor='+statement_descriptor+'&customer='+CustomerId+'&metadata[order_id]='+RecordId);
Http con = new Http();
HttpResponse hs = con.send(http);
system.debug('#### '+ hs.getBody());
String response = hs.getBody();
Integer statusCode=hs.getStatusCode();
if(statusCode == 200 || statusCode == 201){
if(response != null){
//parse the response and create a payment record in Salesforce
}
}else{
if(response != ''){
// parse the error message and create a failed payment record in Salesforce
}
}
}
global class CreateChargeRequest {
@InvocableVariable(label='The ID of an existing customer that will be charged in this request.')
global String CustomerId;
@InvocableVariable(label='Amount intended to be collected by this payment.' required=true)
global decimal Amount;
@InvocableVariable(label='Statement Descriptor')
global String statement_descriptor;
@InvocableVariable(label='Description')
global String description;
@InvocableVariable(label='Salesforce Record Id' required=true)
global String RecordId;
}
}